# Nuxt 3 and Pinia
Integrate Pinia as your state management library for your Nuxt 3 application.
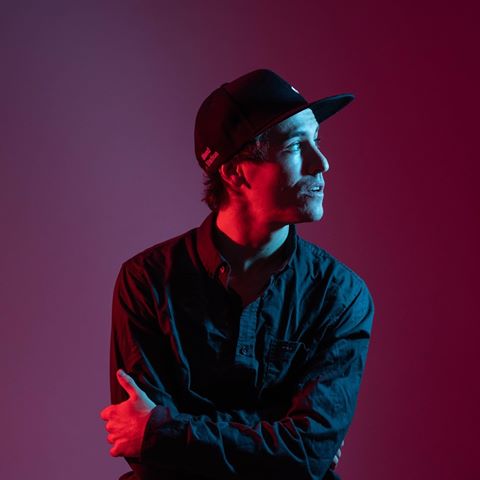
# Vuex -> Pinia
Evan You, the creator of Vue himself, has stated "Pinia is de facto Vuex 5! At this point it’s really a naming/branding issue."
For the time being, it's probably best to be looking towards Pinia content rather than Vuex.
I recommend reading VueJS's official post (opens new window) regarding this to get a better understanding as to why Pinia > Vuex.
# Installing Pinia in Nuxt 3
Pinia nearly comes with first-class support for Nuxt 3. You'll need to install two packages:
yarn add pinia
yarn add @pinia/nuxt
# Add Pinia to your nuxt.config file
You'll need to add '@pinia/nuxt'
to your buildModules array.
// nuxt.config.js
import { defineNuxtConfig } from 'nuxt3'
export default defineNuxtConfig({
buildModules: ['@pinia/nuxt'],
})
# Build your Pinia store
Now build a named store. For my use-case, I needed to manage state regarding filters, so the skeleton of my store looks like:
// store/filters.js
import { defineStore } from 'pinia'
export const useFiltersStore = defineStore({
id: 'filter-store',
state: () => {
return {
filtersList: ['youtube', 'twitch'],
}
},
actions: {},
getters: {
filtersList: state => state.filtersList,
},
})
This is just showing the general structure of your store. The key is to defineStore
and make sure to include an id
. In this case, I'm using 'filter-store'
as my id but it could be anything you prefer.
Read over Pinia's Docs (opens new window) to get a better grasp of how to use Pinia properly.
# Bring Pinia in Vue Component
With our store in place, simply import it into the component you want to use it in and have fun!
<template>
<div>
{{ filtersList }}
</div>
</template>
// components/FilterMenu.vue
<script>
import { useFiltersStore } from '~/store/filters'
export default defineComponent({
setup() {
const filtersStore = useFiltersStore()
const filtersList = filtersStore.filtersList
return { filtersList }
},
})
</script>